LineEdit
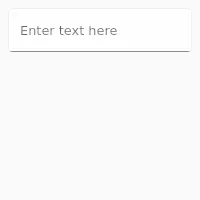
A widget used to enter a single line of text. See TextEdit for a widget able to handle several lines of text.
Properties
Section titled “Properties”enabled
Section titled “enabled” bool default: true
When false, nothing can be entered selecting text is still enabled as well as editing text programmatically.
font-size
Section titled “font-size” length default: 0px
The size of the font of the input text
has-focus
Section titled “has-focus” bool (out)
default: false
Set to true when the line edit currently has the focus
horizontal-alignment
Section titled “horizontal-alignment” enum TextHorizontalAlignment default: left
TextHorizontalAlignment
This enum describes the different types of alignment of text along the horizontal axis of a Text
element.
left
: The text will be aligned with the left edge of the containing box.center
: The text will be horizontally centered within the containing box.right
: The text will be aligned to the right of the containing box.
The horizontal alignment of the text.
input-type
Section titled “input-type” enum InputType default: text
InputType
This enum is used to define the type of the input field.
text
: The default value. This will render all characters normallypassword
: This will render all characters with a character that defaults to ”*”number
: This will only accept and render number characters (0-9)decimal
: This will accept and render characters if it’s valid part of a decimal
The way to allow special input viewing properties such as password fields.
LineEdit { input-type: password;}
placeholder-text
Section titled “placeholder-text” string default: ""
A placeholder text being shown when there is no text in the edit field
read-only
Section titled “read-only” bool default: false
When set to true, text editing via keyboard and mouse is disabled but selecting text is still enabled as well as editing text programmatically.
Functions
Section titled “Functions”focus()
Section titled “focus()”Call this function to focus the LineEdit and make it receive future keyboard events.
clear-focus()
Section titled “clear-focus()”Call this function to remove keyboard focus from this LineEdit
if it currently has the focus. See also FocusHandling.
set-selection-offsets(int, int)
Section titled “set-selection-offsets(int, int)”Selects the text between two UTF-8 offsets.
select-all()
Section titled “select-all()”Selects all text.
clear-selection()
Section titled “clear-selection()”Clears the selection.
copy()
Section titled “copy()”Copies the selected text to the clipboard.
Copies the selected text to the clipboard and removes it from the editable area.
paste()
Section titled “paste()”Pastes the text content of the clipboard at the cursor position.
Callbacks
Section titled “Callbacks”accepted(string)
Section titled “accepted(string)”Invoked when the enter key is pressed.
LineEdit { accepted(text) => { debug("Accepted: ", text); }}
edited(string)
Section titled “edited(string)”Emitted when the text has changed because the user modified it
LineEdit { edited(text) => { debug("Text edited: ", text); }}
key-pressed(KeyEvent) -> EventResult
Section titled “key-pressed(KeyEvent) -> EventResult”Invoked when a key is pressed, the argument is a KeyEvent struct. Use this callback to
handle keys before LineEdit
does. Return accept
to indicate that you’ve handled the event, or return
reject
to let LineEdit
handle it.
key-released(KeyEvent) -> EventResult
Section titled “key-released(KeyEvent) -> EventResult”Invoked when a key is released, the argument is a KeyEvent struct. Use this callback to
handle keys before LineEdit
does. Return accept
to indicate that you’ve handled the event, or return
reject
to let LineEdit
handle it.
© 2025 SixtyFPS GmbH