Common Properties & Callbacks
The Slint elements have many common properties, callbacks and behavior. This page describes these properties and their usage.
Common Visual Properties
Section titled “Common Visual Properties”These properties are valid on all visual items. For example Rectangle
, Text
, and layouts
. Non
visual items such as Timer
don’t have these properties.
length default: 0px
The position of the element relative to its parent.
float default: 0.0
Allows to specify a different order to stack the items with its siblings. The value must be a compile time constant.
width, height
Section titled “width, height” length default: 0px
The width and height of the element. When set, this overrides the default size.
opacity
Section titled “opacity”component ImageInfo inherits Rectangle { in property <float> img-opacity: 1.0; background: transparent; VerticalLayout { spacing: 5px; Image { source: @image-url("elements/slint-logo.png"); opacity: img-opacity; } Text { text: "opacity: " + img-opacity; color: white; horizontal-alignment: center; } }}export component Example inherits Window { width: 100px; height: 310px; background: transparent; Rectangle { background: #141414df; border-radius: 10px; } VerticalLayout { spacing: 15px; padding-top: 10px; padding-bottom: 10px; ImageInfo { img-opacity: 1.0; } ImageInfo { img-opacity: 0.6; } ImageInfo { img-opacity: 0.3; } }}
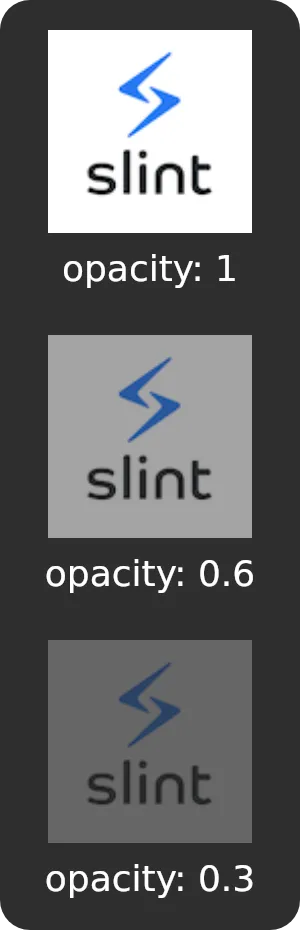
float default: 1
A value between 0 and 1 (or a percentage) that is used to draw the element and its children with transparency. 0 is fully transparent (invisible), and 1 is fully opaque. The opacity is applied to the tree of child elements as if they were first drawn into an intermediate layer, and then the whole layer is rendered with this opacity.
The following example demonstrates the opacity property with children. Note the software renderer does not support layer opacity and this will result in a different end result as shown.
visible
Section titled “visible” bool default: true
When set to false
, the element and all his children won’t be drawn and not react to mouse input. The element
will still take up layout space within any layout container.
absolute-position
Section titled “absolute-position” struct Point (out)
default: a struct with all default values
Point
This structure represents a point with x and y coordinate
x
(length):y
(length):
A common issue is that in a UI with many nested components it’s useful to know their (x,y)position relative to the main window or screen. This convenience property gives easy read only access to that value.
It represents a point specifying the absolute position within the enclosing Window or PopupWindow. It defines coordinates (x,y) relative to the enclosing Window or PopupWindow, but the reference frame is unspecified (could be screen, window, or popup coordinates).
Miscellaneous
Section titled “Miscellaneous”cache-rendering-hint
Section titled “cache-rendering-hint” bool default: false
When set to true
, this provides a hint to the renderer to cache the contents of the element
and all the children into an intermediate cached layer. For complex sub-trees that rarely
change this may speed up the rendering, at the expense of increased memory consumption. Not
all rendering backends support this, so this is merely a hint.
dialog-button-role
Section titled “dialog-button-role” enum DialogButtonRole default: none
DialogButtonRole
This enum represents the value of the dialog-button-role
property which can be added to
any element within a Dialog
to put that item in the button row, and its exact position
depends on the role and the platform.
none
: This isn’t a button meant to go into the bottom rowaccept
: This is the role of the main button to click to accept the dialog. e.g. “Ok” or “Yes”reject
: This is the role of the main button to click to reject the dialog. e.g. “Cancel” or “No”apply
: This is the role of the “Apply” buttonreset
: This is the role of the “Reset” buttonhelp
: This is the role of the “Help” buttonaction
: This is the role of any other button that performs another action.
Specify that this is a button in a Dialog
.
Common Callbacks
Section titled “Common Callbacks”init()
Section titled “init()”Every element implicitly declares an init
callback. You can assign a code block to it that will be invoked when the
element is instantiated and after all properties are initialized with the value of their final binding. The order of
invocation is from inside to outside. The following example will print “first”, then “second”, and then “third”:
component MyButton inherits Rectangle { in-out property <string> text: "Initial"; init => { // If `text` is queried here, it will have the value "Hello". debug("first"); }}
component MyCheckBox inherits Rectangle { init => { debug("second"); }}
export component MyWindow inherits Window { MyButton { text: "Hello"; init => { debug("third"); } } MyCheckBox { }}
Don’t use this callback to initialize properties, because this violates the declarative principle.
Even though the init
callback exists on all components, it cannot be set from application code,
i.e. an on_init
function does not exist in the generated code. This is because the callback is invoked during the creation of the component, before you could call on_init
to actually set it.
While the init
callback can invoke other callbacks, e.g. one defined in a global
section, and
you can bind these in the backend, this doesn’t work for statically-created components, including
the window itself, because you need an instance to set the globals binding. But it is possible
to use this for dynamically created components (for example ones behind an if
):
export global SystemService { // This callback can be implemented in native code using the Slint API callback ensure_service_running();}
component MySystemButton inherits Rectangle { init => { SystemService.ensure_service_running(); } // ...}
export component AppWindow inherits Window { in property <bool> show-button: false;
// MySystemButton isn't initialized at first, only when show-button is set to true. // At that point, its init callback will call ensure_service_running() if show-button : MySystemButton {}}
Accessibility Properties
Section titled “Accessibility Properties”Use the following accessible-
properties to make your items interact well with software like screen readers, braille terminals and other software to make your application accessible.
accessible-role
must be set in order to be able to set any other accessible property or callback.
accessible-role
Section titled “accessible-role” enum AccessibleRole default: the first enum value
AccessibleRole
This enum represents the different values for the accessible-role
property, used to describe the
role of an element in the context of assistive technology such as screen readers.
none
: The element isn’t accessible.button
: The element is aButton
or behaves like one.checkbox
: The element is aCheckBox
or behaves like one.combobox
: The element is aComboBox
or behaves like one.groupbox
: The element is aGroupBox
or behaves like one.image
: The element is anImage
or behaves like one. This is automatically applied toImage
elements.list
: The element is aListView
or behaves like one.slider
: The element is aSlider
or behaves like one.spinbox
: The element is aSpinBox
or behaves like one.tab
: The element is aTab
or behaves like one.tab-list
: The element is similar to the tab bar in aTabWidget
.tab-panel
: The element is a container for tab content.text
: The role for aText
element. This is automatically applied toText
elements.table
: The role for aTableView
or behaves like one.tree
: The role for a TreeView or behaves like one. (Not provided yet)progress-indicator
: The element is aProgressIndicator
or behaves like one.text-input
: The role for widget with editable text such as aLineEdit
or aTextEdit
. This is automatically applied toTextInput
elements.switch
: The element is aSwitch
or behaves like one.list-item
: The element is an item in aListView
.
The role of the element. This property is mandatory to be able to use any other accessible properties. It should be set to a constant value. (default value: none
for most elements, but text
for the Text element)
accessible-checkable
Section titled “accessible-checkable” bool default: false
Whether the element is can be checked or not.
accessible-checked
Section titled “accessible-checked” bool default: false
Whether the element is checked or not. This maps to the “checked” state of checkboxes, radio buttons, and other widgets.
accessible-description
Section titled “accessible-description” string default: ""
The description for the current element.
accessible-enabled
Section titled “accessible-enabled” bool default: false
Whether the element is enabled or not. This maps to the “enabled” state of most widgets. (default value: true
)
accessible-expandable
Section titled “accessible-expandable” bool default: false
Whether the element can be expanded or not. For example, a ComboBox
widget should set this to true,
as its selection can be changed via an expandable popup.
accessible-expanded
Section titled “accessible-expanded” bool default: false
Whether the element is expanded or not. Applies to combo boxes, menu items, tree view items and other widgets.
accessible-label
Section titled “accessible-label” string default: ""
The label for an interactive element. (default value: empty for most elements, or the value of the text
property for Text elements)
accessible-value-maximum
Section titled “accessible-value-maximum” float default: 0.0
The maximum value of the item. This is used for example by spin boxes.
accessible-value-minimum
Section titled “accessible-value-minimum” float default: 0.0
The minimum value of the item.
accessible-value-step
Section titled “accessible-value-step” float default: 0.0
The smallest increment or decrement by which the current value can change. This corresponds to the step by which a handle on a slider can be dragged.
accessible-value
Section titled “accessible-value” string default: ""
The current value of the item.
accessible-placeholder-text
Section titled “accessible-placeholder-text” string default: ""
A placeholder text to use when the item’s value is empty. Applies to text elements.
accessible-read-only
Section titled “accessible-read-only” bool default: false
Whether the element’s content can be edited. This maps to the “read-only” state of line edit and text edit widgets.
accessible-item-selectable
Section titled “accessible-item-selectable” bool default: false
Whether the element can be selected or not.
accessible-item-selected
Section titled “accessible-item-selected” bool default: false
Whether the element is selected or not. This maps to the “is-selected” state of listview items.
accessible-item-index
Section titled “accessible-item-index” int default: 0
The index (starting from 0) of this element in a group of similar elements. Applies to list items, radio buttons and other elements.
accessible-item-count
Section titled “accessible-item-count” int default: 0
The total number of elements in a group. Applies to the parent container of a group of element such as list views, radio button groups or other grouping elements.
Accessibility Callbacks
Section titled “Accessibility Callbacks”You can also use the following callbacks that are going to be called by the accessibility framework:
accessible-action-default()
Section titled “accessible-action-default()”Invoked when the default action for this widget is requested (eg: pressed for a button).
accessible-action-set-value(string)
Section titled “accessible-action-set-value(string)”Invoked when the user wants to change the accessible value.
accessible-action-increment()
Section titled “accessible-action-increment()”Invoked when the user requests to increment the value.
accessible-action-decrement()
Section titled “accessible-action-decrement()”Invoked when the user requests to decrement the value.
accessible-action-expand()
Section titled “accessible-action-expand()”Invoked when the user requests to expand the widget (eg: disclose the list of available choices for a combo box).
© 2025 SixtyFPS GmbH